A contour is a closed curve joining all the continuous points having some color or intensity, they represent the shapes of objects found in an image. Contour detection is a useful technique for shape analysis and object detection and recognition.
In a previous tutorial, we have discussed about edge detection using Canny algorithm and we've seen how to implement it in OpenCV, you may ask, what's the difference between edge detection and contour detection ?
Well, when we perform edge detection, we find the points where the intensity of colors changes significantly and then we simply turn those pixels on. However, contours are abstract collections of points and segments corresponding to the shapes of the objects in the image. As a result, we can manipulate contours in our program such as counting number of contours, using them to categorize the shapes of objects, cropping objects from an image (image segmentation) and much more.
Contour detection is not the only algorithm for image segmentation though, there are a lot others, such as the current state-of-the-art semantic segmentation, hough transform and K-Means segmentation.
For a better accuracy, here is the pipeline we gonna follow to successfully detect contours in images:
- Convert the image to a binary image, it is a common practice for the input image to be a binary image (which should be a result of a thresholded image or edge detection).
- Finding the contours using findContours() OpenCV function.
- Draw these contours and show the image.
Alright, let's get started. First, let's install the dependencies for this tutorial:
pip3 install matplotlib opencv-python
Importing the necessary modules:
import cv2
import matplotlib.pyplot as plt
We gonna use this image for this tutorial:
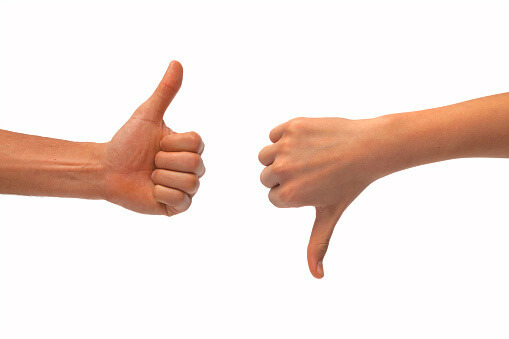
Let's load it:
# read the image
image = cv2.imread("thumbs_up_down.jpg")
Converting it to RGB and gray scale:
# convert to RGB
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_RGB2GRAY)
As mentioned earlier in this tutorial, we gonna need to create a binary image, which means each pixel of the image is either black or white. This is a necessary in OpenCV, finding contours is like finding white object from black background, objects to be found should be white and the background should be black.
# create a binary thresholded image
_, binary = cv2.threshold(gray, 225, 255, cv2.THRESH_BINARY_INV)
# show it
plt.imshow(binary, cmap="gray")
plt.show()
The above code creates the binary image by eliminating (setting to 0) pixels which has a value of less than 225 and turning on (setting to 255) the pixels which has a value of more than 225, here is the output image:
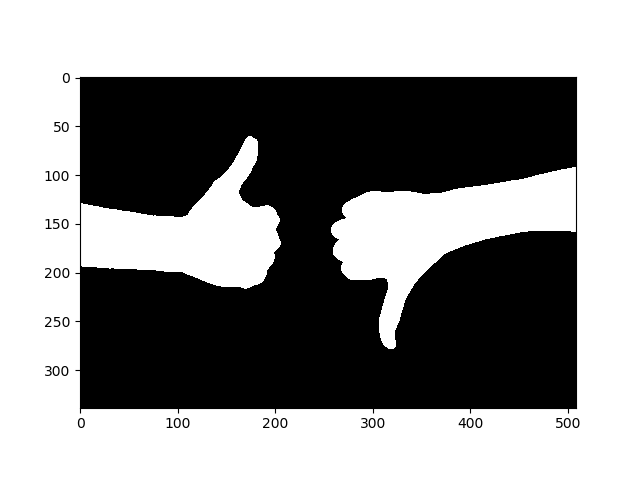
Now this is easy for OpenCV to detect contours:
# find the contours from the thresholded image
contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# draw all contours
image = cv2.drawContours(image, contours, -1, (0, 255, 0), 2)
The above code finds contours within the binary image and draw them with a thick green line to the image, let's show it:
# show the image with the drawn contours
plt.imshow(image)
plt.show()
Output image:
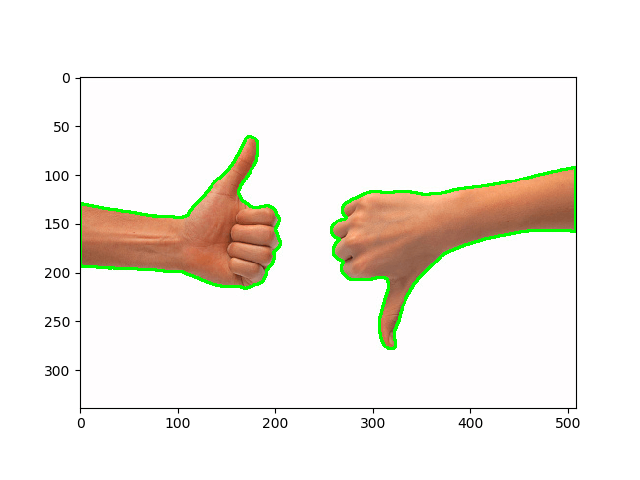
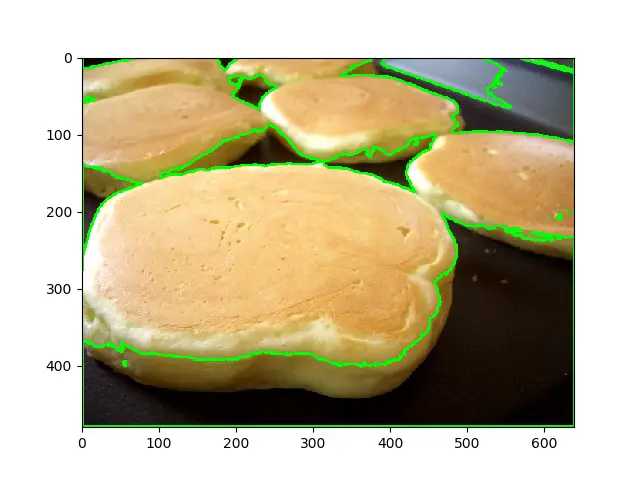
Please check OpenCV's official documentation for more information.
Code for How to Detect Contours in Images using OpenCV in Python
You can also view the full code on github.
contour_detector.py
import cv2
import matplotlib.pyplot as plt
# read the image
image = cv2.imread("thumbs_up_down.jpg")
# convert to RGB
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_RGB2GRAY)
# create a binary thresholded image
_, binary = cv2.threshold(gray, 225, 255, cv2.THRESH_BINARY_INV)
# show it
plt.imshow(binary, cmap="gray")
plt.show()
# find the contours from the thresholded image
contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# draw all contours
image = cv2.drawContours(image, contours, -1, (0, 255, 0), 2)
# show the image with the drawn contours
plt.imshow(image)
plt.show()
live-contour-detector.py
import cv2
cap = cv2.VideoCapture(0)
while True:
_, frame = cap.read()
# convert to grayscale
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# create a binary thresholded image
_, binary = cv2.threshold(gray, 255 // 2, 255, cv2.THRESH_BINARY_INV)
# find the contours from the thresholded image
contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# draw all contours
image = cv2.drawContours(frame, contours, -1, (0, 255, 0), 2)
# show the images
cv2.imshow("gray", gray)
cv2.imshow("image", image)
cv2.imshow("binary", binary)
if cv2.waitKey(1) == ord("q"):
break
cap.release()
cv2.destroyAllWindows()
0 comments:
Post a Comment